Web-plugin Authorization
After your web-plugin is installed, it is available in the team and to all team members. Before the web-plugin can access any board content or open windows, each team member must first authorize the web-plugin and grant the required permissions. To check and request authorization, you'll need two methods: miro.isAuthorized
and miro.requestAuthorization
. Here's an example of how to use these methods:
async function onToolbarAppButtonClicked () {
const isAuthorized = await miro.isAuthorized()
if (!isAuthorized) {
// Ask the user to authorize the app.
await miro.requestAuthorization()
}
// Once authorized, open the app.
openMyApp()
}
function openMyApp () {
miro.board.ui.openLeftSidebar('main-app-sidebar.html')
}
Authorization of apps using the Miro REST API
If your app uses both the client SDK and the Miro REST API, you’ll need to provide extra parameters to miro.requestAuthorization
to ensure that the user's access credentials are passed to your application backend for storage and future use:
async function onToolbarAppButtonClicked () {
const isAuthorized = await miro.isAuthorized()
if (isAuthorized) {
// Open the app.
openMyApp()
} else {
// State is an optional parameter.
// You can use it to pass extra parameters to the redirect URI page or for security purposes.
// See https://developers.google.com/identity/protocols/oauth2/web-server#creatingclient,
const installState = await fetch(
'https://my-app-server.com/api/install-state',
{ credentials: 'same-origin' }
).then(res => res.text())
// Ask the user to authorize the app.
await miro.requestAuthorization({
// To successfully complete the miro.requestAuthorization call,
// the redirect URI page must redirect the user back to Miro.
// See the documentation below.
redirect_uri: 'https://my-app-server.com/install',
state: installState
})
// Once authorized, open the app.
openMyApp()
}
}
function openMyApp () {
miro.board.ui.openLeftSidebar('main-app-sidebar.html')
}
Once the app's backend has successfully completed the authorization flow for the Miro REST API, the redirect URI page must redirect the user back to the following Miro page:
https://miro.com/app-install-completed/?client_id=YOUR_APPLICATION_ID&team_id=ID_OF_THE_TEAM_THE_APP_HAS_BEEN_INSTALLED_TO
Where:
The client_id
is the Client id on the Miro App Settings page. App dashboard →
The team_id
is the team_id from the JSON response containing the non-expiring access_token. For more information, see Getting an access token.
Testing the authorization for your web-plugin during development
To verify the authorization for your web-plugin:
- Go to the team app settings.
- Find your app and uninstall the app only for yourself (not for the whole team).
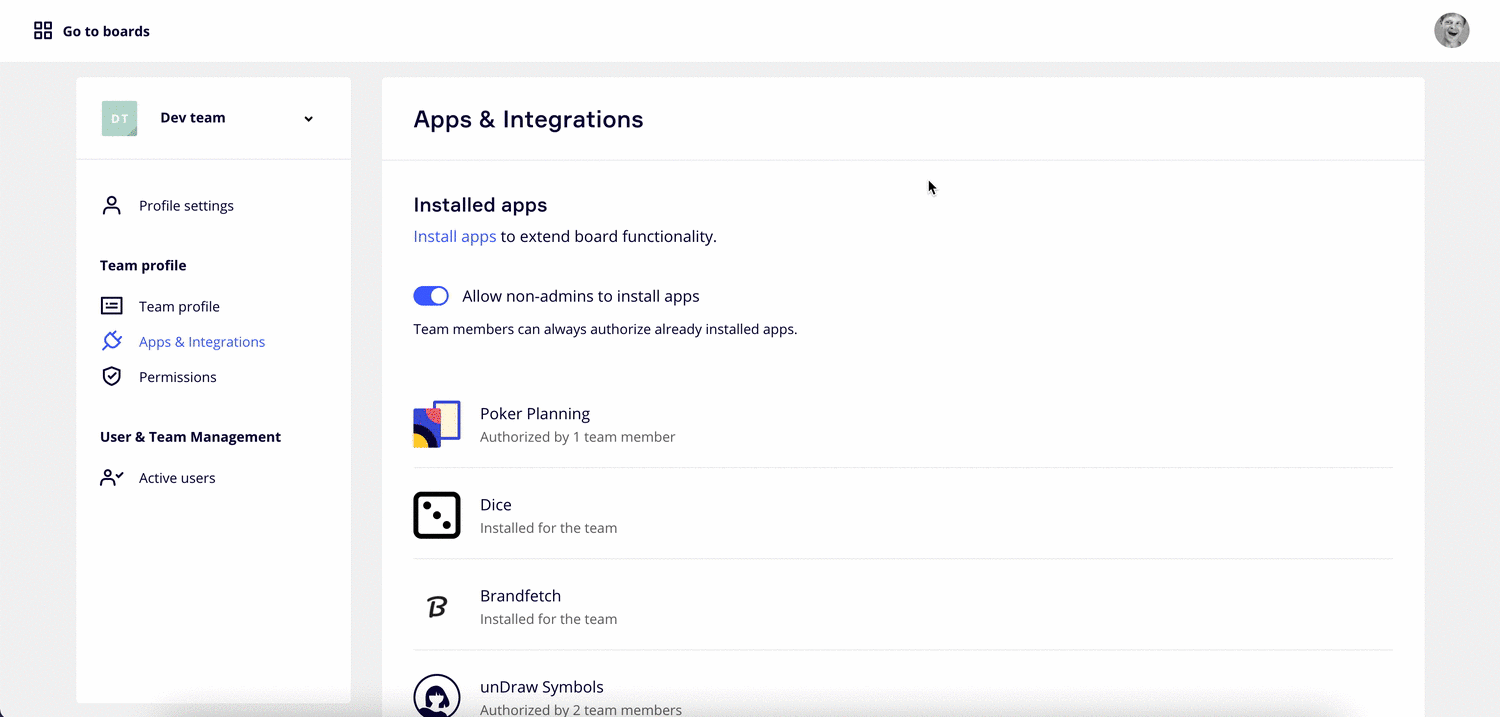
- Open a board within the same team.
- Use your app to verify that the app checks and requests authorization.
Updated over 3 years ago