Collaboration
Experimental
The collaboration
namespace provides methods and components to handle collaborative features on Miro boards, including: sessions
, attention management
, and remote viewport control
.
Sessions are the foundational component necessary for leveraging collaborative features. It is necessary to start a collaborative session (sessionStart) in order to manage features like attention management and remote viewport control.
miro.board.collaboration
provides methods and components that allow apps to create collaborative experiences across multiple users on the same board. This includes controlling the viewport, attention management capabilities such as zooming into user content, and more.
- startSession: starts a collaborative session.
- getSessions: retrieves existing sessions for the current app.
- zoomTo: zooms the specified user to one or more items on the board.
- on: subscribes to different collaborative events.
- off: unsubscribes from previously subscribed collaborative events.
- attention: provides access to methods related to managing attention.
-
- follow: initiates the process of following a specified online user(s), referred to as the followed user.
- unfollow: is used to stop a user(s) from following another user.
- isFollowing: checks whether the current user is already following another user on the board.
- getFollowedUser: if a user is following another user, the method returns the followed user details.
Methods
startSession(...)
(props: SessionStartProps) => Promise<Session>
🚦 Rate limit: Level 1
🔒 Requires scope: boards:write
An application can start and manage collaborative sessions for participants on a board through the miro.board.collaboration
namespace. In order to implement collaborative features, such as setting the focus and viewport control of other users on a board, an application must first start an active session.
Concurrent sessions
Applications support the ability to run multiple active sessions at one time on a board (for example, breakout rooms). Only one application can run active, concurrent sessions on a board at a time. Participants on a board can only be in one unique session at a time.
ℹ️ Note: Once you start the session, you get a session object returned, with this object you can perform further operations, such as ending the session.
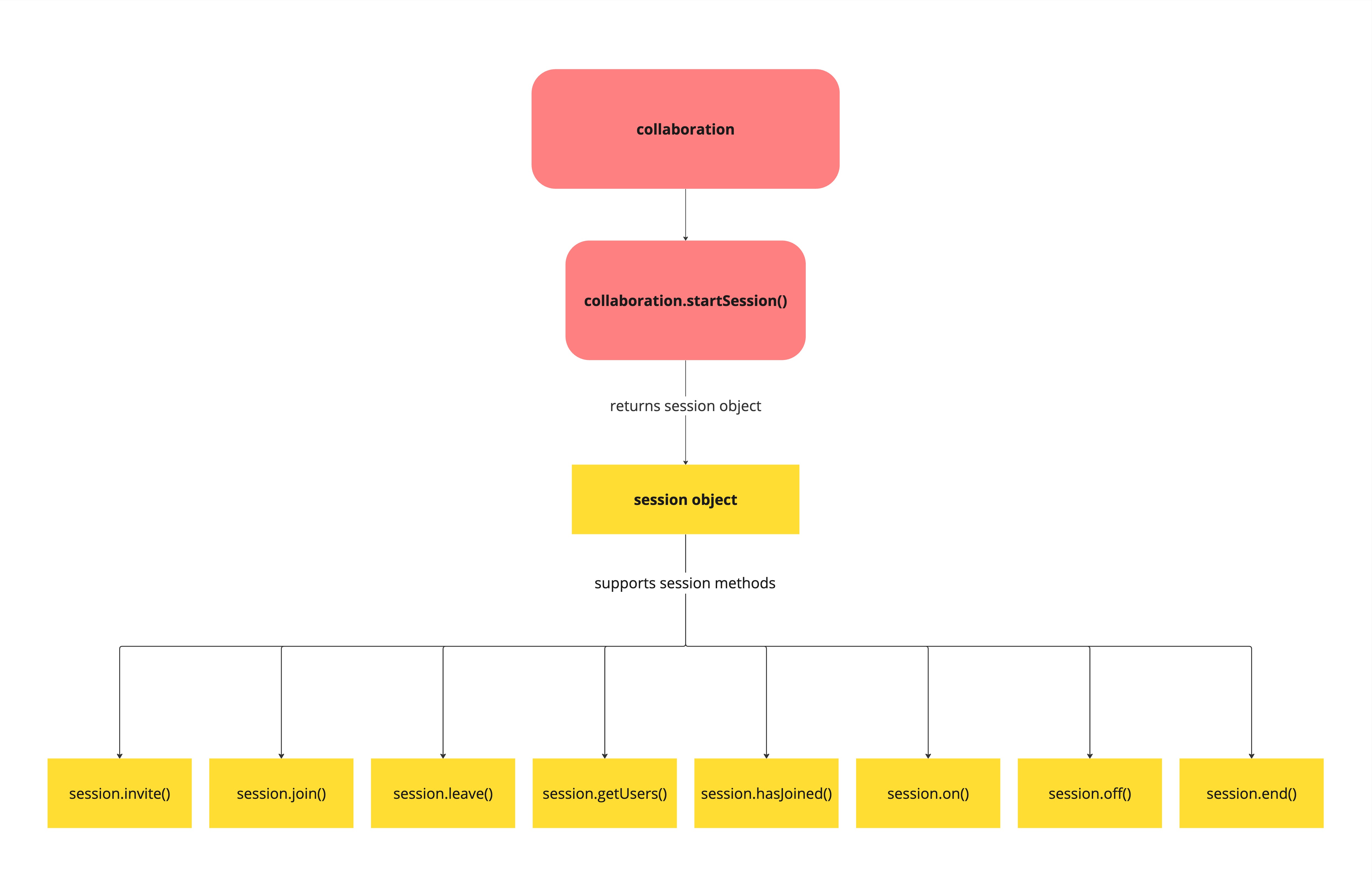
sessionStart creates, starts and returns a Session for the current app.
sessionStart accepts an object with the following properties:
All properties
Property | Type |
---|---|
color? |
string |
description? |
string |
name |
string |
name
defines the name of the session that will be displayed in some parts of the UI.
color
is an optional parameter that accepts a hexadecimal color which can also be used in some parts of the UI.
description
is an optional parameter that will be used in some places in the UI.
getSessions(...)
() => Promise<Array<Session>>
🚦 Rate limit: Level 1
🔒 Requires scope: boards:read
The getSessions returns a list of the active Sessions for the current application.
zoomTo(...)
( user: OnlineUserInfo, items: OneOrMany<{ connectorIds?: readonly Array<string> createdAt: readonly string createdBy: readonly string groupId?: readonly string title="id">id: readonly string linkedTo?: string modifiedAt: readonly string modifiedBy: readonly string origin: Origin parentId: readonly string | 'null' relativeTo: RelativeTo title="type">type: string title="x">x: number title="y">y: number bringInFrontOf: (target: BaseItem) => Promise<void> bringToFront: () => Promise<void> getConnectors: () => Promise<Array<Connector>> getLayerIndex: () => Promise<number> getMetadata: (key: string) => Promise<T> goToLink: () => Promise<boolean> sendBehindOf: (target: BaseItem) => Promise<void> sendToBack: () => Promise<void> setMetadata: (key: string, value: title="Json">Json) => Promise<T> title="sync">sync: () => Promise<void> }> ) => Promise<void>
🚦 Rate limit: Level 1
🔒 Requires scope: boards:read
zoomTo
method zooms the specified user to one or more items on the board. If you zoom to items that are scattered across the board, the method can zoom out to include all the items passed as arguments in the viewport.
This method will throw an error if:
- No user and items are passed as arguments.
- The specified user is not online.
- The specified user is not in some session of your app.
Example:
// Get currently online users.
const onlineUsers = await miro.board.getOnlineUsers();
// Retrieve the user that you want to navigate.
const user = onlineUsers.find((user) => user.name.includes("Sara"));
// Create three sticky notes
const first = await miro.board.createStickyNote({
content: 'first',
x: 400,
y: 400,
});
const second = await miro.board.createStickyNote({
content: 'second',
x: -400,
y: -400,
});
const third = await miro.board.createStickyNote({
content: 'third',
});
// Zoom to a sticky note on the board
await miro.board.collaboration.zoomTo(user, first);
// Or: zoom to multiple sticky notes on the board
await miro.board.collaboration.zoomTo(user, [second, third]);
// Or: zoom to all sticky notes on the board
const stickyNotes = await miro.board.get({
type: 'sticky_note',
});
await miro.board.collaboration.zoomTo(user, stickyNotes);
on(...)
(name: 'sessions:started' | 'sessions:ended', handler: (event: SessionsLifecycleEvent) => void) => Promise<void>
Subscribes to collaboration events in your app
sessions:started
: Triggered when any session in your app has started.sessions:ended
: Triggered when any session in your app has ended.
Both sessions:Started
and sessions:Ended
will receive a SessionsLifecycleEvent object as a parameter.
SessionsLifecycleEvent
Property | Type |
---|---|
session |
{ color: readonly string description: readonly string title="id">id: readonly string title="name">name: readonly string starterId: readonly string starterName: readonly string end: () => Promise<void> getUsers: () => Promise<Array<string>> hasJoined: (user: string) => Promise<boolean> invite: (users: Array<OnlineUserInfo> | Array<Array<OnlineUserInfo>>) => Promise<void> join: () => Promise<void> leave: () => Promise<void> title="off">off: (title="name">name: 'user-joined', handler: (event: UserSessionEvent) => Promise<void>) => Promise<void> title="on">on: (title="name">name: 'user-joined', handler: (event: UserSessionEvent) => Promise<void>) => Promise<void> } |
Example:
// Registers to the 'sessions:started' event emitted by the same app when a session has started
miro.board.collaboration.on('sessions:started', async (event: SessionsLifecycleEvent) => {
const { session } = event
});
const session = await miro.board.collaboration.startSession({
name: 'My session'
})
off(...)
(name: 'sessions:started' | 'sessions:ended', handler: (event: SessionsLifecycleEvent) => void) => Promise<void>
Unsubscribes from previously subscribed collaboration events in your app
sessions:started
: Triggered when any session in your app has started.sessions:ended
: Triggered when any session in your app has ended.
Example:
const handler = async (event: SessionsLifecycleEvent)) => {
const { session } = event
}
// Registers to the 'sessions:started' event emitted by the same app when a session has started
miro.board.collaboration.on('sessions:started', handler);
// Unsubscribe from the 'sessions:started' event handler.
miro.board.collaboration.off('sessions:started', handler);
const session = await miro.board.collaboration.startSession({
name: 'My session'
})
All properties
Property | Type |
---|---|
attention |
readonly { follow: (followee: OnlineUserInfo, options?: FollowUserSessionsOptions) => Promise<void> getFollowedUser: () => Promise<OnlineUserInfo> isFollowing: () => Promise<boolean> unfollow: (options?: UnfollowUserSessionsOptions) => Promise<void> } |
getSessions(...) |
() => Promise<Array<Session>> |
off(...) |
(title="name">name: 'sessions:started' | 'sessions:ended', handler: (event: SessionsLifecycleEvent) => void) => Promise<void> |
on(...) |
(title="name">name: 'sessions:started' | 'sessions:ended', handler: (event: SessionsLifecycleEvent) => void) => Promise<void> |
startSession(...) |
(props: SessionStartProps) => Promise<Session> |
zoomTo(...) |
( user: OnlineUserInfo, items: OneOrMany<{ connectorIds?: readonly Array<string> createdAt: readonly string createdBy: readonly string groupId?: readonly string title="id">id: readonly string linkedTo?: string modifiedAt: readonly string modifiedBy: readonly string origin: Origin parentId: readonly string | 'null' relativeTo: RelativeTo title="type">type: string title="x">x: number title="y">y: number bringInFrontOf: (target: BaseItem) => Promise<void> bringToFront: () => Promise<void> getConnectors: () => Promise<Array<Connector>> getLayerIndex: () => Promise<number> getMetadata: (key: string) => Promise<T> goToLink: () => Promise<boolean> sendBehindOf: (target: BaseItem) => Promise<void> sendToBack: () => Promise<void> setMetadata: (key: string, value: title="Json">Json) => Promise<T> title="sync">sync: () => Promise<void> }> ) => Promise<void> |
Updated 5 months ago